How to secure gRPC APIs
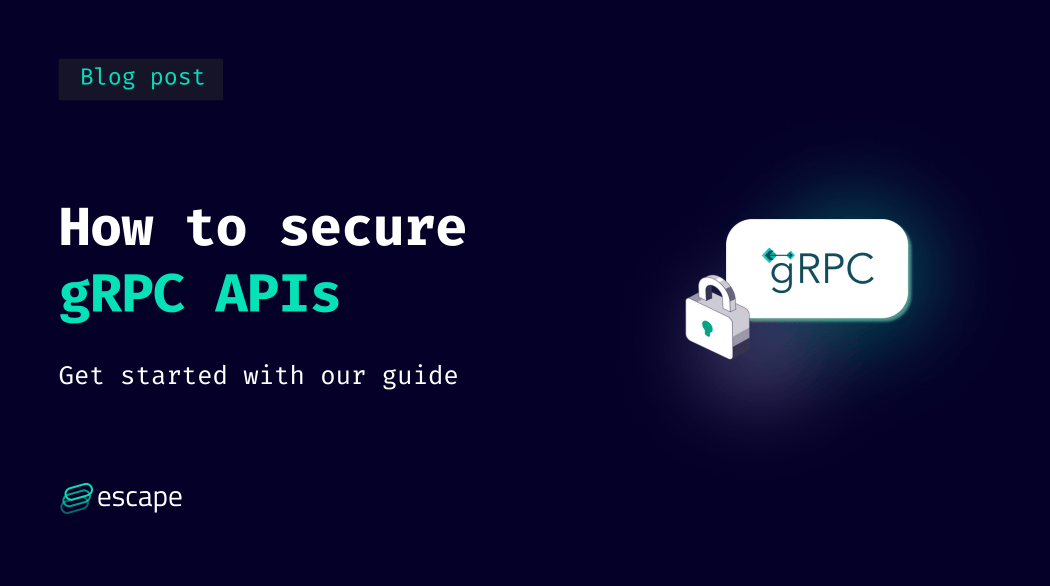
Looking for ways to secure gRPC APIs? Don't worry, we've got you covered!
In this article, we will be exploring the topic of gRPC security and its importance in ensuring the security of API endpoints. We will also discuss the role of dynamic application security testing (DAST) tools in securing gRPC APIs and identifying and fixing vulnerabilities before they reach production.
What is gRPC?
gRPC is a high-performance, open-source RPC (Remote Procedure Call) framework developed by Google. It enables efficient communication between distributed systems by allowing clients and servers to call methods on each other as if they were local objects, while handling complexities such as serialization, authentication, and load balancing. gRPC leverages HTTP/2 for transport, Protocol Buffers for serialization, and supports multiple programming languages, making it an efficient and versatile solution for building distributed systems.
What makes gRPC better than REST?
In summary, gRPC offers numerous advantages over REST, with its superior performance being a standout feature.
By using Protocol Buffers for data transmission, gRPC achieves lower latency and higher throughput, making it ideal for real-time applications and large data transfers. Check out this article to find out how faster it is (the larger the payload, the more noticeable the benefit of protobuf vs JSON)
Additionally, gRPC's strongly typed contracts ensure consistency between client and server, facilitating easier maintenance and evolution of APIs over time. Its support for bidirectional streaming and HTTP/2 further enhance communication efficiency, while code generation simplifies development and interoperability across multiple programming languages promotes flexibility.
Moreover, gRPC's advanced error handling capabilities, flow control, and cancellation features contribute to building more reliable and scalable applications in distributed systems, marking it as a preferred choice for modern software development.
GRPC is also used within Escape's internal microservices and plays a crucial role in communicating between different components of the platform. By leveraging GRPC, we ensure efficient and secure communication between its services.
The challenges of gRPC security testing
While GRPC offers many benefits, it also presents unique challenges when it comes to security testing.
First, traditional security scanners are often designed to work with REST APIs and may not have built-in support for gRPC. This can make it difficult to effectively test the security of gRPC services.
Another challenge is understanding the schema of a gRPC application. Unlike REST APIs, which often have well-documented schemas like OpenAPI or Swagger, gRPC applications traditionally lacked a standardized schema format.
However, with the introduction of "server reflection" in gRPC, this has become less of an issue.
Server reflection in gRPC provides a mechanism for clients to query the server for its supported services, methods, and message types dynamically. It serves as an equivalent to introspection in GraphQL or the schema in OpenAPI.
This feature allows clients to discover and understand the capabilities of a gRPC server dynamically, facilitating easier integration and development. However, it's important to note that server reflection needs to be enabled and properly configured on the server side for clients to utilize this functionality effectively.
Why test gRPC APIs?
Testing gRPC services is crucial for ensuring their reliability, functionality, and performance. Through thorough testing, developers can identify and rectify potential issues early in the development lifecycle, ultimately leading to the delivery of high-quality software products. Here are several reasons why testing gRPC services is essential:
- Testing gRPC services ensures reliability, functionality, and performance.
- Early testing helps identify and fix potential issues in the development lifecycle.
- Verification of service correctness and adherence to defined contracts is facilitated.
- Compatibility with client applications can be validated through testing.
- Rigorous testing enhances confidence in service behavior and fosters trust among users.
- Overall, testing contributes to the delivery of high-quality software products.
Common gRPC vulnerabilities
Injection attacks in gRPC
gRPC services are often connected to various components, and this interconnectedness can expose them to injection attacks. When we talk about injection attacks in the context of gRPC services, we typically refer to scenarios where untrusted data is improperly handled, allowing attackers to inject malicious code or unexpected input into the system. This can lead to various security vulnerabilities, such as SQL injection, command injection, or even code injection.
Let's consider an example of how injection vulnerabilities can manifest in a gRPC service:
Suppose we have a gRPC service that accepts user input to construct and execute a database query. The service might look something like this:
service DatabaseService {
rpc QueryDatabase (QueryRequest) returns (QueryResponse) {}
}
message QueryRequest {
string query = 1;
}
message QueryResponse {
repeated string results = 1;
}
And the corresponding server implementation might look like this (written in a hypothetical programming language):
class DatabaseServiceServicer(DatabaseServiceServicer):
def QueryDatabase(self, request, context):
query = request.query
# Execute the database query
results = execute_query(query)
return QueryResponse(results=results)
In this example, the QueryDatabase
method accepts a query string from the client and directly executes it against the database. This approach is highly vulnerable to injection attacks, such as SQL injection, where an attacker could craft a malicious SQL query and inject it into the query
parameter.
To mitigate injection vulnerabilities, developers should adopt practices such as parameterized queries, input validation, and proper sanitization of user input. Here's how we could improve the above code to prevent SQL injection:
import psycopg2
class DatabaseServiceServicer(DatabaseServiceServicer):
def QueryDatabase(self, request, context):
query = request.query
# Use parameterized queries to prevent SQL injection
conn = psycopg2.connect(database="my_database", user="my_user", password="my_password", host="localhost", port="5432")
cursor = conn.cursor()
cursor.execute("SELECT * FROM my_table WHERE column = %s", (query,))
results = cursor.fetchall()
cursor.close()
conn.close()
return QueryResponse(results=results)
In this improved version, we're using parameterized queries with placeholders (%s
) instead of directly interpolating user input into the SQL query string. This approach prevents SQL injection by separating the query logic from the data, ensuring that user input is treated as data rather than executable code.
Additionally, insecure communication channels and insufficient input validation can also lead to vulnerabilities in GRPC services.
To mitigate this problem, you can use protovalidate - a series of libraries designed to validate Protobuf messages at runtime based on user-defined validation rules. It helps you to enforce requirements like minimum and maximum lengths or allowed patterns using regular expressions for string
, bool
, and bytes
fields.
To integrate validation rules into your gRPC service, you need to define the User
message with the specified validation rules in your .proto
file. Here's how you can add it:
syntax = "proto3";
import "buf/validate/validate.proto";
message User {
string name = 1 [(buf.validate.field).string.min_len = 1, (buf.validate.field).string.max_len = 100];
string email = 2 [(buf.validate.field).string.email = true];
bool verified = 3 [(buf.validate.field).bool.const = true];
bytes password = 4 [(buf.validate.field).bytes.pattern = "^[a-zA-Z0-9]*$"];
}
service YourService {
// Define your service methods here
}
In this example, the User
message contains four fields: name
, email
, verified
, and password
.
- The
name
field is required to have a minimum length of 1 and a maximum length of 100 characters. - The
email
field must be a valid email address. - The
verified
field must always be set to true. - The
password
field must match the specified regular expression pattern, which allows only alphanumeric characters.
After defining the User
message with the validation rules, you can use it in your gRPC service methods as needed. When clients send requests containing User
objects, the gRPC server will automatically validate the fields based on the specified rules. If any field fails validation, gRPC will return an error response to the client.
By adopting secure coding practices and carefully validating and sanitizing input data, developers can effectively mitigate injection vulnerabilities in their gRPC services.
Vulnerabilities related to Personally Identifiable Information (PII) in gRPC
Vulnerabilities related to Personally Identifiable Information (PII) in gRPC services can pose significant risks to data privacy and security. Let's explore some common scenarios and potential vulnerabilities related to PII, along with code examples:
Insecure data transmission:
When sensitive PII data is transmitted over insecure channels without proper encryption, it can be intercepted by attackers. This vulnerability can be mitigated by using secure communication protocols like TLS/SSL. Here's an example of how to configure gRPC with TLS encryption:
// Define gRPC service with TLS encryption
service MySecureService {
rpc TransmitSensitiveData (SensitiveDataRequest) returns (SensitiveDataResponse) {}
}
message SensitiveDataRequest {
string pii_data = 1;
}
message SensitiveDataResponse {
string response = 1;
}
And the corresponding server implementation in Python using TLS:
import grpc
from grpc import ssl_channel_credentials
class MySecureServiceServicer(MySecureServiceServicer):
def TransmitSensitiveData(self, request, context):
pii_data = request.pii_data
# Process sensitive data securely
response = process_sensitive_data(pii_data)
return SensitiveDataResponse(response=response)
server = grpc.server(ssl_channel_credentials())
add_MySecureServiceServicer_to_server(MySecureServiceServicer(), server)
server.add_secure_port('[::]:50051')
server.start()
Insecure Data Storage:
Storing PII data on servers without proper encryption or access controls can lead to data breaches. Ensure that PII data is encrypted at rest and access is restricted to authorized personnel only. Here's an example using Google Cloud Key Management Service (KMS) for encryption:
from google.cloud import kms_v1
def encrypt_data(data):
# Encrypt PII data using Google Cloud KMS
kms_client = kms_v1.KeyManagementServiceClient()
# Replace 'key_ring_name', 'key_name', and 'location' with actual values
name = kms_client.crypto_key_path_path('project_id', 'location', 'key_ring_name', 'key_name')
response = kms_client.encrypt(name, data.encode('utf-8'))
return response.ciphertext
def decrypt_data(encrypted_data):
# Decrypt PII data using Google Cloud KMS
kms_client = kms_v1.KeyManagementServiceClient()
# Replace 'key_ring_name', 'key_name', and 'location' with actual values
name = kms_client.crypto_key_path_path('project_id', 'location', 'key_ring_name', 'key_name')
response = kms_client.decrypt(name, encrypted_data)
return response.plaintext.decode('utf-8')
Logging Sensitivity:
Logging PII data without proper masking or obfuscation can expose sensitive information. Ensure that log messages are appropriately sanitized before being written to logs. Here's an example using Python's logging module with sensitive data masking:
It is crucial for developers to follow secure coding practices and regularly test and scan their GRPC applications to identify and mitigate these vulnerabilities.
import logging
def log_sensitive_data(pii_data):
# Log sensitive data with masking
masked_data = mask_sensitive_data(pii_data)
logging.info("Received sensitive data: %s", masked_data)
def mask_sensitive_data(pii_data):
# Mask sensitive data (e.g., replace with asterisks)
return "*" * len(pii_data)
By addressing these vulnerabilities with appropriate security measures, developers can mitigate the risks associated with handling PII data in gRPC services.
Insecure authentication and authorization
Another common vulnerability in gRPC is insecure authentication and authorization mechanisms. If not properly implemented, attackers can bypass authentication and gain unauthorized access to sensitive data or perform malicious actions.
To define them in your proto file:
// Define authentication and authorization rules in the proto file
service YourService {
rpc YourMethod (YourRequest) returns (YourResponse) {
option (google.api.http) = {
get: "/your-method"
body: "*"
};
option (grpc.gateway.protoc_gen_openapiv2.options.openapiv2_operation) = {
security: {
custom_auth: {
// Define authentication mechanism here
authorization_header: "Authorization"
// Add more authentication configurations if needed
}
}
};
}
}
This code defines how the gRPC service method YourMethod
should be exposed over HTTP, including specifying how authentication should be handled for this method.
The gRPC API security scanner with custom security tests
Traditionally, security scanners have not been able to scan gRPC services effectively. However, Escape - the API security platform powered by AI-enhanced DAST, has developed a scanner that can test gRPC services directly. This scanner overcomes the challenges posed by gRPC's efficient communication protocol, allowing engineers to identify vulnerabilities in gRPC services.
Escape's gRPC API security scanner leverages the capabilities of the in-house algorithm to overcome the challenge of understanding the schema of a gRPC application.
Escape's DAST scanner also supports custom security tests, allowing security engineers to specify values for their APIs during scanning. This feature enables thorough testing of different business logic branches within the application. You can check out our documentation on custom tests to learn more.
To perform a scan, the application must be up and running. Escape's DAST scanner communicates with the application and sends malicious requests to identify vulnerabilities (i.e. black box penetration testing). The scan time depends on the size and complexity of the application. Escape provides a user-friendly platform that presents the scan results in a human-readable format, making it easy for engineers to interpret the findings and use detailed remediation code snippets to fix their issues.
The future of gRPC security
With the increasing adoption of gRPC, it is essential to have robust security measures in place to protect sensitive data and ensure the integrity of applications. With Escape, engineers can shift security left and ensure the security of their applications before they reach production.
If you have any questions or would like to learn more about Escape's gRPC security solutions, feel free to reach out to our team. We are here to help you secure your applications and protect your data.
💡Want to learn more?
Check out the following articles: