10 best practices to secure your Spring Boot applications
Explore the top 10 Spring Boot security best practices from the Escape team to secure your Java web applications efficiently.
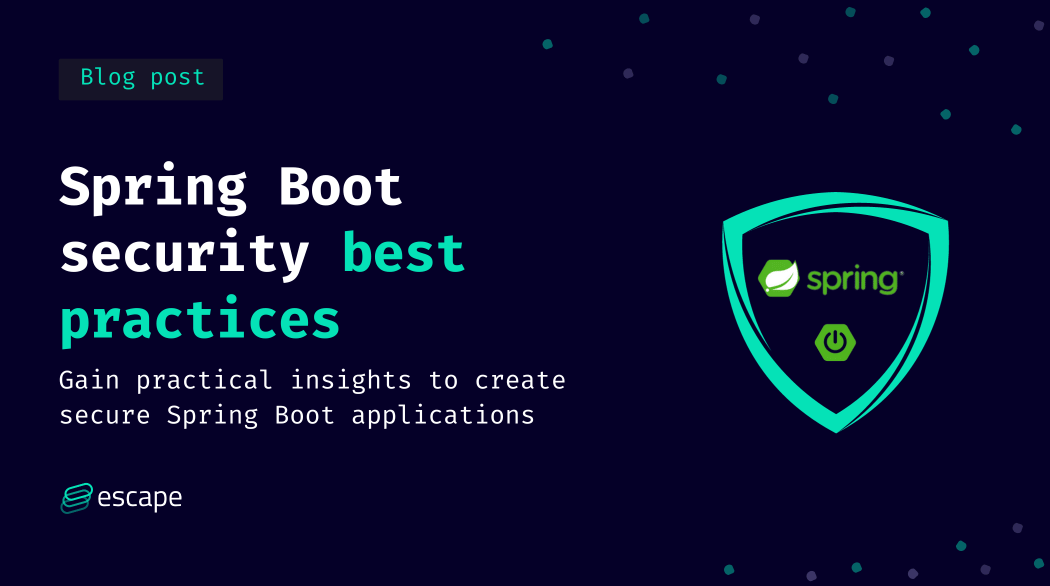
Wondering how to enhance the security of your Spring Boot applications?
In our latest blog post,'10 Best Practices to Secure Your Spring Boot Applications,''we present practical insights from the Escape team. Discover actionable tips to secure your Java web applications and reap the benefits of a more secure development environment.
What is Spring Boot?
Spring Boot is an open-source, Java-based framework that uses minimal effort to create stand-alone, production-grade Java web applications. It builds on the powerful Spring framework, making it more accessible and easier to use.
Why Spring Boot needs to be secured?
Spring Boot is widely used in the enterprise, especially in industries like banking and financial services. The exploitation of vulnerabilities might happen, as seen with an exploit for a zero-day remote code execution vulnerability in the Spring Framework dubbed 'Spring4Shell' in 2022 and might present a severe implications for organizations.
Confirmed! https://t.co/olfK9dEr30
— Janggggg (@testanull) March 30, 2022
More than that, while the vulnerability had specific requirements to be exploited, even sample code from spring.io was vulnerable:
Can confirm! The #Spring4Shell exploit in the wild appears to work against the stock "Handling Form Submission" sample code from https://t.co/dt05rTPbGQ
— Will Dormann (@wdormann) March 31, 2022
If the sample code is vulnerable, then I suspect there are indeed real-world apps out there that are vulnerable to RCE... https://t.co/PFXoIusFcT pic.twitter.com/2gydOJk10Y
Developers often use sample code as a blueprint for their applications, potentially resulting in numerous vulnerable apps available online.
So, the need for security measures is rooted in the following key aspects:
- Sensitive data handling: Many Spring Boot-based applications deal with sensitive information, including personal and financial data. This makes them attractive targets for malicious actors seeking to exploit vulnerabilities and gain unauthorized access to valuable data.
- Regulatory compliance: Industries like finance are subject to strict regulatory requirements, such as GDPR, HIPAA, or PCI DSS. Ensuring the security of Spring Boot applications is essential to meeting these compliance standards and avoiding legal repercussions or financial penalties.
- Risk of data breaches: Securing Spring Boot applications is crucial for preventing unauthorized access to confidential data and averting potentially severe consequences, including reputational damage.
- Availability and reliability: Spring Boot applications often serve critical functions within enterprise ecosystems. Any compromise in security could lead to service disruptions, impacting business operations and causing financial losses.
- Maintaining customer trust: In sectors like finance, where customer trust is paramount, the security of applications is directly linked to the reputation of the organization. A security breach not only jeopardizes data but erodes customer confidence.
- Adhering to best practices: Secure coding practices are integral to the software development lifecycle. Implementing security measures in Spring Boot applications aligns with industry best practices, fostering a security-first mindset among developers and contributing to the overall maturity of the software development process.
In this post, the Escape security research team has detailed 10 critical security best practices for Spring Boot applications, adding practical code examples to assist developers in implementation.
Best practices for Spring Boot security
1. Implement HTTPS
HTTPS encrypts data during transmission, preventing unauthorized parties from intercepting and deciphering sensitive information. This is particularly vital for applications dealing with user credentials, financial transactions, or any other confidential data. So, secure communication is non-negotiable. You must us HTTPS to protect data in transit.
In your application.properties
, ensure TLS/SSL is enabled:
server.port=8443
server.ssl.key-store=classpath:keystore.jks
server.ssl.key-store-password=yourpassword
server.ssl.key-password=yourpassword
Adjust the file paths and make sure to replace "keystore.jks," "key-store-password," and "key-password" with your actual keystore file, keystore password, and private key password.
If you want to enforce HTTPS and disallow HTTP access entirely, you can modify the Spring Security configuration to redirect all HTTP traffic to HTTPS. Here's how you can achieve this:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.requiresChannel()
.requestMatchers(r -> r.getHeader("X-Forwarded-Proto") != null)
.requiresSecure()
.and()
.authorizeRequests()
.antMatchers("/").permitAll() // Allow access to the home page
.anyRequest().authenticated()
.and()
.formLogin().and()
.httpBasic().and()
.csrf().and(); // Retaining CSRF configuration
}
.requiresChannel().requestMatchers(r -> r.getHeader("X-Forwarded-Proto") != null).requiresSecure()
enforces HTTPS for all requests. The requestMatchers
part is included to handle cases where your application is behind a proxy or load balancer, and X-Forwarded-Proto
is used to determine the protocol.
If you want to enforce HTTPS using WebSecurityConfigurerAdapter
in a Spring Boot application, you can configure security settings to ensure a secure connection. Below is a complete example:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.requiresChannel()
.requestMatchers(r -> r.getHeader("X-Forwarded-Proto") != null)
.requiresSecure() // Enforce HTTPS
.and()
.authorizeRequests()
.antMatchers("/").permitAll() // Allow access to the home page
.anyRequest().authenticated()
.and()
.formLogin().and()
.httpBasic().and()
.csrf().and(); // Retain CSRF protection
}
}
Thoroughly test your application after implementing HTTPS to ensure seamless functionality. Regularly monitor the SSL/TLS configuration for any vulnerabilities or expired certificates.
2. Activate CSRF protection
Cross-Site Request Forgery (CSRF) attacks can be devastating. Spring Security enables CSRF protection by default. Verify it's not mistakenly disabled:
http
.csrf().disable(); // Avoid this in production
3. Validate input rigorously
Never trust user input. Always validate for type, length, format, and range. Use Spring's built-in validation:
import javax.validation.constraints.NotEmpty;
public class UserInput {
@NotEmpty(message = "Name cannot be empty")
private String name;
// getters and setters
}
4. Use parameterized queries
SQL injections are very critical vulnerabilities that are common in web applications and can allow attackers to completely take over the application's database.
In Spring Boot, SQL Injection attacks can be mitigated by using parameterized queries or JPA repositories:
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT u FROM User u WHERE u.email = :email")
User findByEmail(@Param("email") String email);
}
5. Enable method level security
Method level authorization problems happen when an unauthorized or low-privileged user can access the sensitive functions of an application like functions normally scoped to administrator roles.
This issue is so well spread among web applications that it has become a part of OWASP's Top 10 vulnerabilities in web applications.
You can avoid this security issue by restricting access at the method level using Spring Security annotations:
@PreAuthorize("hasRole('ADMIN')")
public void deleteUser(Long id) {
// deletion logic
}
6. Encrypt sensitive data
Want to avoid data leaks? Sensitive data should be encrypted. Use Spring's @EncryptablePropertySource
for property file encryption:
@EncryptablePropertySource(name = "EncryptedProperties", value = "classpath:encrypted.properties")
@Configuration
public class EncryptionConfig {
// Configuration details
}
7. Regularly update dependencies
Spring Boot vulnerabilities often appear in third-party packages and plugins. Keep dependencies up-to-date to download the patches created by the developers and ensure known security vulnerabilities are fixed. Use tools like Maven or Gradle for easy management.
More recently, open-source dependency scanning tools like Trivy have added support for scanning vulnerable Java dependencies.
8. Implement proper authentication and authorization
Utilize Spring Security's robust authentication and authorization mechanisms. Configure authentication providers, userDetailsService, and password encoders:
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth
.userDetailsService(userDetailsService)
.passwordEncoder(passwordEncoder());
}
9. Audit and log security events
Security audits are essential to properly assess the security posture of an organization's applications. We strongly advice to keep track of all security-related events. Spring Boot Actuator and Spring Security's audit capabilities are useful:
public class CustomAuditListener extends AbstractAuthenticationAuditListener {
@Override
public void onApplicationEvent(AbstractAuthenticationEvent event) {
// Logging logic
}
}
10. Conduct security testing
Regularly test your application for security vulnerabilities.
You can write your tests using Spring Boot's own testing capabilities but this approach can be time-consuming, especially since you need to create and maintain all the test cases for many types of security bugs.
@SpringBootTest
public class SecurityConfigurationTest {
// Test cases
}
Conclusion
Security in Spring Boot is not an afterthought but a critical aspect of application design. By following these best practices, developers can significantly improve the security posture of their Spring Boot applications.
A faster and more reliable alternative to manual assessments is to use a dedicated API security testing tool with Spring Boot support like Escape DAST, which can scan all your exposed API endpoints within minutes, test even for complex business logic vulnerabilities and help developers remediate any issues.
Secure your Spring Boot applications with Escape DAST
Meet your compliance mandates quickly, reduce the load on your developers, and remediate vulnerabilities more effectively than ever
Get a demo with our product expertWant to learn more about framework security?💡