ASP.NET security best practices
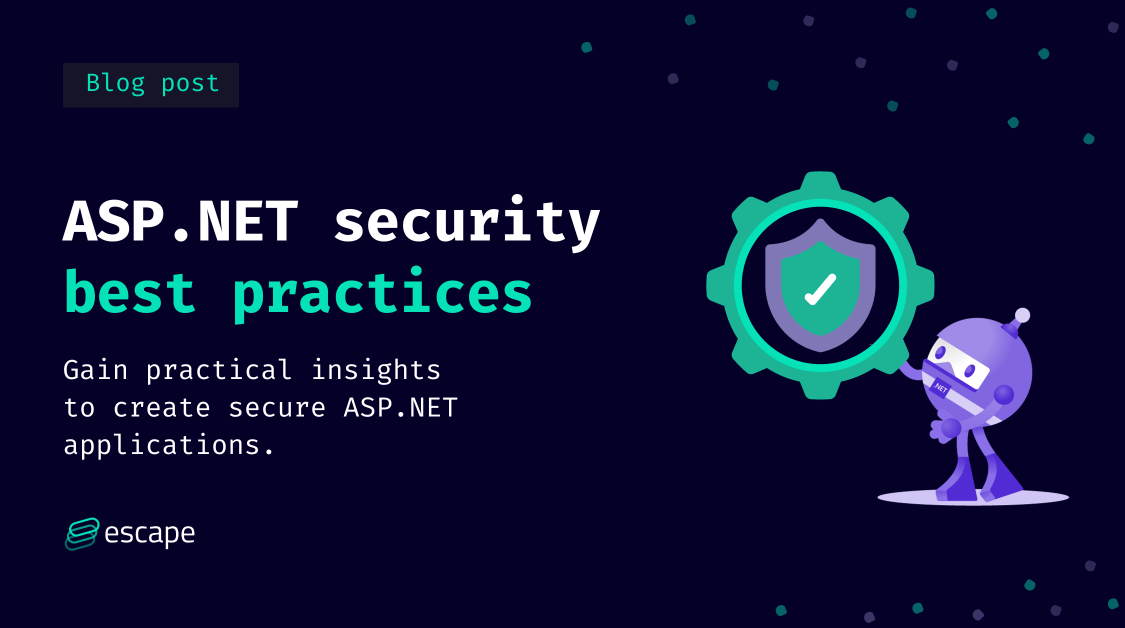
Curious about the key strategies to ensure the security and reliability of your ASP.NET applications, including building APIs? Dive into our latest blog post, where we guide you through ASP.NET security best practices. Explore how these practices can not only enhance the security of your web applications but also bring tangible benefits to your development journey.
In this guide, Escape's security research team has gathered 10 crucial ASP.NET security best practices that empower you to create more resilient and efficient ASP.NET projects. Let's get started!
What is ASP.NET?
ASP.Net core is a popular framework for creating web applications and APIs using Microsoft's .NET runtime.
Due to their usage in many enterprise companies, ASP.NET applications often handle sensitive data or have strong requirements for availability. In this context, best practices for security are not just a feature; they are a necessity.
ASP.NET security best practices:
1. Enforce HTTPS
Why it's important
Using HTTPS ensures that the data between your server and the client is encrypted, making it more difficult for attackers to intercept or tamper with data.
How to implement
In your ASP.NET application, configure the RequireHttpsAttribute
in your Global.asax
file:
protected void Application_Start()
{
GlobalFilters.Filters.Add(new RequireHttpsAttribute());
}
2. Use Anti-Forgery Tokens
Why it's important
CSRF attacks exploit the trust that a web application has in an authenticated user's browser, allowing attackers to perform unauthorized actions on behalf of the user. For instance, if a user is authenticated in one tab of their browser and visits a malicious site in another, the malicious site can send requests to the authenticated site without the user's knowledge. These requests can change user settings, post content, or even initiate transactions.
Anti-forgery tokens prevent Cross-Site Request Forgery (CSRF) attacks by ensuring that the requests sent to your server are from legitimate users.
How to implement
In your Razor views, use the Html.AntiForgeryToken()
method:
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<!-- Form content goes here -->
}
3. Validate input
Why it's important
Input validation is a cornerstone of web application security, particularly in ASP.NET applications.
The importance of this practice comes from its role in defending against various forms of injection attacks, such as SQL injection, Cross-Site Scripting (XSS), and others. When user input is not properly validated or sanitized, attackers can exploit these vulnerabilities to inject malicious code into your system.
Consequences from the exploitation of injection vulnerabilities can be as disastrous as having your whole database erased by attackers. This is why, at Escape, we also created a specific set of security tests to help you find and fix injections in your applications.
How to implement
Use ASP.NET's built-in validation controls or data annotations:
[Required]
[Display(Name = "Username")]
public string UserName { get; set; }
4. Implement Authentication and Authorization
Why it's important
Strong authentication and authorization ensure that only legitimate users can access certain parts of your application.
Authentication issues are so well spread among web applications that they have become a part of OWASP's Top 10 vulnerabilities in web applications.
How to implement
You can use ASP.NET Identity for implementing role-based security in your ASP.NET applications:
[Authorize(Roles = "Admin")]
public ActionResult AdminOnly()
{
return View();
}
5. Secure Session Management
Why it's important
Secure session management is vital to protect user data and prevent unauthorized access.
Each of your user sessions contains sensitive information, such as user preferences, authentication tokens, and other personal data.
If this session data is not properly secured, it can be vulnerable to attacks like session hijacking or fixation, where attackers gain control of a user's session and thereby access their account and associated privileges. This can lead to data breaches, identity theft, and unauthorized actions performed on behalf of the user.
Implementing secure session management practices, such as using secure, HTTP-only cookies and storing session data on the server side, significantly reduces these risks. It ensures that session tokens are not easily intercepted or tampered with, maintaining the integrity and confidentiality of user sessions.
How to implement
Store sensitive information in server-side sessions and use secure, HTTP-only cookies.
Session["UserId"] = user.Id;
6. Encrypt configuration files
Why it's important
Encrypting configuration files in ASP.NET is a critical security measure.
Configuration files often contain sensitive data, such as database connection strings and API keys, which are essential for the application's operations.
If these files are left unencrypted, they become an easy target for attackers who gain access to the file system. Once compromised, this sensitive information can be used to launch further attacks, access databases, and misuse API services.
Encrypting these files adds a robust layer of security, making it much harder for unauthorized individuals to decipher the contents even if they gain access to the files. This practice is a straightforward yet effective way to safeguard important credentials and configuration details, ensuring they remain confidential and secure.
How to implement
Use the aspnet_regiis.exe
tool to encrypt sections of your Web.config
file.
For example, to encrypt the connectionStrings section of an application located at /MyApp, use:
aspnet_regiis -pe "connectionStrings" -app "/MyApp"
7. Implement error handling
Why it's important
When an application encounters an error, the default behavior might be to display a detailed error message. These messages can include sensitive information such as stack traces, database queries, or internal system details, which could be invaluable to a potential attacker.
By exposing such information, the application becomes vulnerable to various attacks, including SQL injection, system exploitation, and more.
Detailed error messages can also lead to poor user experience, causing confusion and mistrust. Implementing custom error handling not only prevents the leakage of sensitive information but also allows for a more controlled and user-friendly response to unexpected situations.
This approach ensures that users see a standardized error page that doesn't give away any internal workings of the application, thus maintaining both security and professionalism.
How to implement
Use custom error pages and avoid detailed error messages in production:
<customErrors mode="On" defaultRedirect="ErrorPage.html"/>
8. Regularly update framework and libraries
Why it's important
Regularly updating the framework and libraries in ASP.NET applications is a key security practice.
This is because updates often include patches for known vulnerabilities.
As security threats evolve and new vulnerabilities are discovered, the developers of these frameworks and libraries release updates to address these issues. By not updating, you leave your application exposed to known vulnerabilities, which attackers can easily exploit.
How to implement
Regularly check for and install updates to ASP.NET and third-party libraries. OWASP Dependency check can help you find and update outdated dependencies in your ASP.NET projects.
9. Logging and monitoring
Why it's important
Logging and monitoring are essential components in the security infrastructure of any web application.
They are important for your security team to detect and respond to security incidents.
By maintaining comprehensive logs, you create a detailed record of all activities and events occurring within your application. This information is invaluable when it comes to understanding the context and scope of any security breach. In the event of an attack or a security anomaly, these logs can be analyzed to pinpoint how the incident occurred, what vulnerabilities were exploited, and what parts of the system were affected.
Even more important, proactive monitoring of these logs enables you to identify and respond to potential security threats in real-time. It acts as an early warning system, alerting you to unusual or suspicious activities that could indicate a security breach or an attempted attack.
How to implement
Implement logging using libraries like NLog or Serilog and regularly monitor logs for suspicious activities.
10. Perform proper security testing
Why it's important
Most critical security bugs can only be found through proper security testing and assessment.
Adding security tests as part of your regular software testing process is critical.
How to implement
You can use .NET testing frameworks like Nunit to write your custom security tests.
using NUnit.Framework;
using Moq;
using System.Web.Mvc;
using MyApplication.Controllers;
using System.Security.Principal;
using System.Web;
using System.Web.Routing;
namespace MyApplication.Tests
{
[TestFixture]
public class SecureControllerTests
{
[Test]
public void TestAdminOnlyAction_AccessedByAdmin_ReturnsView()
{
// Write test and assertions here
}
}
}
but this approach can be time-consuming, especially since you need to create and maintain all the test cases for many types of security bugs.
A faster and more reliable alternative is to use a dedicated API security testing tool with ASP.NET support like Escape, which will test your application against 100+ security best practices and vulnerabilities directly in the CI/CD, and help you remediate all issues.
Conclusion
Securing an ASP.NET application requires a combination of best practices. By following these guidelines, developers can significantly enhance the security posture of their applications, ensuring a safer experience for all users.
However, keep in mind that security is a continuous process, and modern shift-left standards recommend a DevSecOps approach with security integrated at all stages of the development processes.
💡 Want to learn more about framework security? We've got you covered:
- 9 GraphQL Security Best Practices
- Top 7 DevSecOps Best Practices
- API security checklist
- What are Insecure Direct Object References (IDOR) in GraphQL, and how to fix them
FAQ
Why is ASP.NET security crucial for web applications?
ASP.NET security is essential to protect web applications from unauthorized access, data breaches, and various cyber threats. Implementing robust security practices ensures the confidentiality, integrity, and availability of your application's data and functionality.
What is the significance of input validation in ASP.NET security?
Input validation is critical in ASP.NET security to prevent common vulnerabilities like SQL injection and cross-site scripting (XSS). By validating and sanitizing user inputs, you reduce the risk of malicious code injection and enhance the overall resilience of your application.
How can I secure sensitive data in transit in ASP.NET applications?
To secure data in transit, use HTTPS to encrypt communication between the web browser and the server. Configuring SSL/TLS ensures that sensitive information, such as login credentials and personal data, is encrypted during transmission, safeguarding it from eavesdropping.
What role does authentication play in ASP.NET security best practices?
Authentication verifies the identity of users accessing your application. Implementing strong authentication mechanisms, such as multi-factor authentication (MFA), ensures that only authorized individuals can interact with your application, adding an extra layer of protection against unauthorized access.
How can ASP.NET developers handle session security effectively?
To enhance session security, developers should use secure session management techniques. This includes using secure cookies, implementing session timeouts, and regenerating session IDs after login. These measures help mitigate the risk of session hijacking and unauthorized access to user sessions.